Flask Full Course | Complete Python Web Development Training Guide
Master Python web development with this Flask full course. Learn routing, templates, databases, APIs, and deployment step-by-step with hands-on Flask projects.
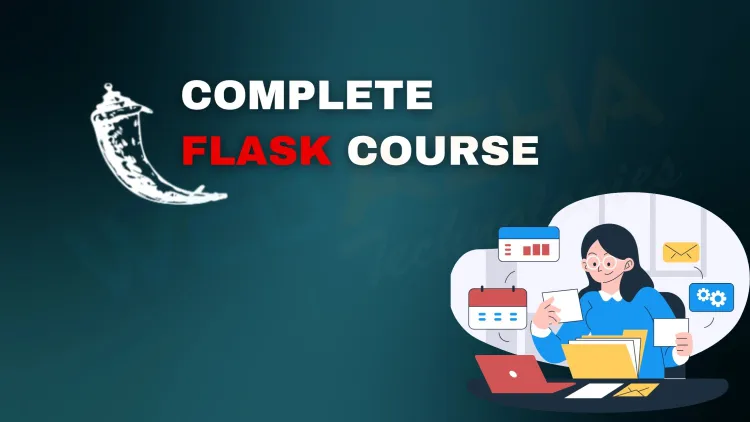
If you're a Python enthusiast looking to dive into web development, Flask is an excellent framework to start with. It’s lightweight, flexible, and beginner-friendly, yet powerful enough to build production-grade applications. In this complete guide, we’ll walk you through the full Flask course curriculum, from the fundamentals to building and deploying real-world projects.
What Is Flask and Why Should You Learn It?
Flask is a micro web framework written in Python. Unlike full-stack frameworks like Django, Flask gives you the freedom to choose your tools and structure. It's minimalistic, making it perfect for beginners who want to understand web development from the ground up.
Key Benefits of Learning Flask:
-
Lightweight and easy to set up
-
Ideal for REST API development
-
Large community and extensions
-
Great for learning how the web works under the hood
-
Widely used in startups and prototyping
Who Is This Flask Course For?
This full Flask course is designed for:
-
Python beginners interested in web development
-
Backend developers who want to build web APIs
-
Frontend developers expanding into full-stack development
-
Data scientists needing to deploy dashboards or models
-
Computer science students and career changers
Prerequisites for Learning Flask
To get the most out of this course, you should have:
-
Basic Python programming knowledge
-
Familiarity with HTML and CSS
-
Optional: Understanding of HTTP, APIs, and JSON
Complete Flask Course Curriculum: What You’ll Learn
Here’s a breakdown of the key topics covered in a comprehensive Flask full course:
1. Introduction to Web Development
-
What is a web framework?
-
Client-server architecture
-
Static vs dynamic websites
2. Setting Up the Flask Environment
-
Installing Python and pip
-
Creating a virtual environment
-
Installing Flask using pip
-
Project structure overview
3. First Flask App
-
Writing your first Flask application
-
Running the development server
-
Flask route and view functions
-
Returning HTML responses
4. Templates with Jinja2
-
Introduction to Jinja templating
-
Using dynamic content in HTML
-
Template inheritance
-
Filters and control structures
5. Working with Forms
-
Handling GET and POST requests
-
HTML forms and Flask form data
-
Using Flask-WTF for form validation
-
CSRF protection and custom validators
6. Flask and Databases
-
Introduction to SQLite and SQLAlchemy
-
Setting up a database connection
-
Creating models with SQLAlchemy
-
Performing CRUD operations
-
Database migrations with Flask-Migrate
7. Flask Blueprints and Application Structure
-
Why use blueprints?
-
Creating modular Flask apps
-
Structuring large-scale applications
8. Authentication and Authorization
-
User registration and login
-
Password hashing with Werkzeug
-
Flask-Login integration
-
Creating protected routes
-
Session management and logout
9. RESTful APIs with Flask
-
Introduction to REST APIs
-
Creating API routes with Flask
-
JSON responses and status codes
-
Using Flask-RESTful or Flask-Classy
-
Building a simple CRUD API
10. AJAX and JavaScript Integration
-
Fetch API and XMLHttpRequest
-
Handling async requests in Flask
-
JSON endpoints and real-time updates
11. File Uploads and Static Files
-
Uploading images and files
-
Saving and retrieving user files
-
Serving static assets (CSS, JS, images)
12. Error Handling and Debugging
-
Custom error pages (404, 500)
-
Logging and debugging tools
-
Flask debugger and error tracking
13. Unit Testing Flask Apps
-
Writing tests with
unittest
orpytest
-
Testing routes and forms
-
Using Flask’s test client
-
Test coverage and automation
14. Security Best Practices
-
Input validation and sanitization
-
SQL injection and XSS prevention
-
HTTPS and secure cookies
-
Using Flask-Talisman for secure headers
15. Deployment and Hosting
-
Preparing your Flask app for production
-
Using Gunicorn and Nginx
-
Deploying on platforms like Heroku, Render, or AWS
-
Environment variables and configuration
Capstone Project: Build and Deploy a Real Flask App
The best way to solidify your Flask knowledge is to build a real-world project. A typical capstone project could include:
-
A blog platform
-
A task manager or to-do app
-
A RESTful API service
-
A personal portfolio site
-
A mini social network
You’ll apply everything you’ve learned: authentication, databases, templates, and API integration — and finally deploy it online.
Advantages of Taking a Full Flask Course
Hands-On Learning
You write actual code as you learn, building projects step-by-step.
Career Opportunities
Flask is used in production systems across startups, fintech, and data science.
Python Compatibility
Flask integrates smoothly with NumPy, pandas, TensorFlow, and other Python libraries.
Open Source Community
Plenty of open-source projects, documentation, and forums for support.
Common Tools and Libraries Used with Flask
Tool/Library | Purpose |
---|---|
Flask-WTF | Form validation |
Flask-Login | Authentication and session management |
SQLAlchemy | ORM for database interaction |
Jinja2 | Templating engine |
Flask-Migrate | Database migrations |
Gunicorn | Production-ready WSGI server |
Flask-Mail | Sending emails from your app |
Flask-CORS | Cross-Origin Resource Sharing setup |
Final Thoughts: Is Learning Flask Worth It?
Absolutely. Flask is a top choice for developers who want to:
-
Understand the full web development cycle
-
Build and deploy apps quickly
-
Work with Python in web environments
-
Develop REST APIs and integrate with frontends or mobile apps
Whether you’re aiming for a software developer job or just want to build your own apps, a full Flask course equips you with the practical skills needed in today’s development landscape.
FAQs
What is the Flask full course about?
The Flask full course is a step-by-step training program designed to teach Python web development using the Flask framework. It covers basics to advanced topics like routing, templates, forms, databases, APIs, and deployment.
Who should take this Flask course?
This course is ideal for Python beginners, web developers, data scientists, or students looking to create web apps and APIs using Flask.
Do I need to know Python before learning Flask?
Yes, basic knowledge of Python is essential before starting a Flask course, as it helps you understand the logic and syntax used in web development.
How long does it take to complete the Flask full course?
On average, learners can complete the course in 4–6 weeks, depending on prior experience and practice time dedicated to projects and exercises.
Is Flask better than Django for beginners?
Yes, Flask is often recommended for beginners due to its minimalistic and flexible nature, allowing learners to understand core web development concepts.
Does this course include real-world projects?
Yes, most full Flask courses include hands-on projects such as blogs, task managers, or APIs to help learners apply their skills in practical scenarios.
Can I build REST APIs with Flask?
Absolutely. Flask is widely used for building RESTful APIs using extensions like Flask-RESTful and Flask-Classy.
Does Flask support database integration?
Yes, Flask supports databases like SQLite and PostgreSQL using SQLAlchemy, an Object Relational Mapper (ORM) for Python.
Is Flask suitable for large applications?
While Flask is minimalistic, it can scale using Blueprints and modular structures, making it suitable for both small and large applications.
How do I deploy a Flask app after completing the course?
You can deploy Flask apps using Gunicorn and Nginx on platforms like Heroku, Render, or AWS. The course typically includes deployment tutorials.
Is Flask good for data science applications?
Yes, Flask is excellent for creating dashboards and APIs to deploy machine learning models in data science projects.
Does this course include authentication features?
Yes, advanced sections in the course usually cover user registration, login, logout, session management, and Flask-Login integration.
Can I use JavaScript with Flask?
Yes, Flask supports front-end integration using JavaScript, AJAX, or frameworks like React or Vue for dynamic behavior.
Is Flask used in industry?
Yes, Flask is popular among startups and companies needing lightweight, customizable web applications or APIs.
Will I learn about templating in this course?
Yes, Jinja2 templating is covered extensively to help you create dynamic and reusable HTML pages.
Is Flask beginner-friendly?
Yes, Flask is highly recommended for Python beginners venturing into web development due to its simple syntax and flexibility.
Does the course explain error handling in Flask?
Yes, proper error handling, custom error pages, and debugging techniques are covered in a complete Flask course.
Can I connect Flask with frontend libraries like Bootstrap?
Yes, Flask easily integrates with Bootstrap and other CSS/JS frameworks to enhance UI/UX.
Do I need a specific IDE to follow the course?
No, any Python-compatible IDE like VS Code, PyCharm, or even Jupyter Notebooks can be used for Flask development.
Is the Flask full course free or paid?
It depends on the platform or training provider. Some offer free YouTube courses, while others provide premium, structured programs.
Does the course include Flask Blueprints?
Yes, Blueprints are included in intermediate to advanced modules to help structure scalable apps.
Are unit testing and security covered?
Yes, topics like Flask testing, debugging, and securing apps against vulnerabilities (like XSS, CSRF) are typically included.
Will I get a certificate after completing the Flask course?
If you take the course through a training provider or platform, a certificate of completion is usually issued.
Can I use Flask for mobile app backends?
Yes, Flask is widely used to create REST APIs for mobile applications, making it backend-compatible for Android and iOS.
How does Flask handle static files?
Flask uses a special /static
folder to serve CSS, JS, and image files efficiently.
What libraries are commonly used with Flask?
Popular libraries include Flask-WTF (forms), Flask-Login (authentication), SQLAlchemy (databases), and Flask-Migrate (migrations).
Is Flask open-source?
Yes, Flask is an open-source project and freely available for use and modification under the BSD license.
Will I learn deployment in the Flask course?
Yes, deployment on cloud platforms like Heroku or AWS using Gunicorn and Nginx is generally part of the curriculum.
Can I build an e-commerce website using Flask?
Yes, with sufficient knowledge, you can build complex apps like e-commerce platforms using Flask and related tools.